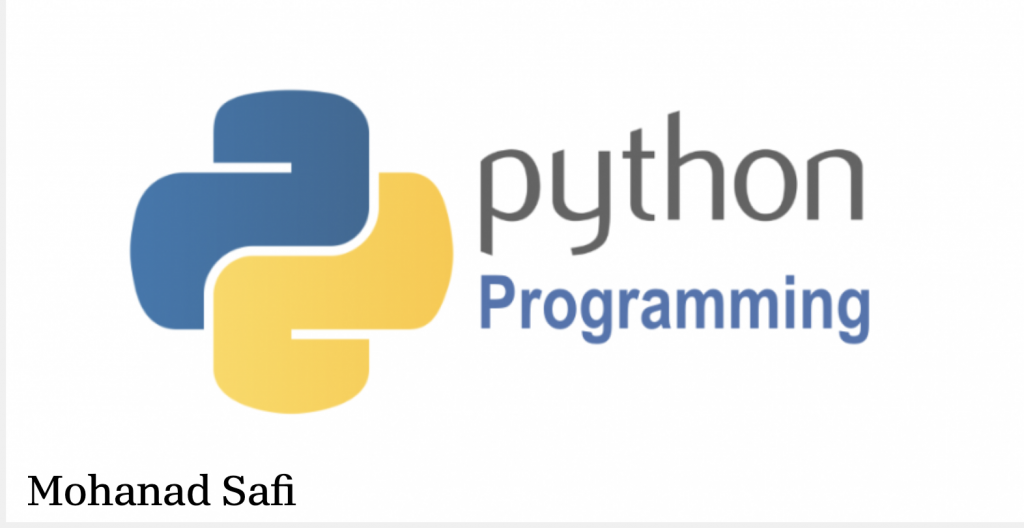
Python Learning for Beginners
Introduction (Page 3): Embark on your programming journey with Python—an essential language known for its versatility and readability. From web development to data science and artificial intelligence, Python is your gateway to the world of coding.
Chapter 1: Getting Started with Python (Page 4): Dive into the coding realm by installing Python and creating your first program. “Hello, World!” sets the stage as you grasp fundamental concepts like variables, data types, and basic input/output.
Chapter 2: Control Flow and Functions (Page 5): Master the art of controlling program flow with conditional statements and loops. Functions become your allies as you organize code efficiently, emphasizing the importance of clarity.
Chapter 3: Data Structures (Page 6+7): Uncover the power of Python’s data structures—lists, tuples, sets, and dictionaries. Learn when and how to use each structure through hands-on examples and exercises.
Chapter 4: File Handling and Modules (Page 8+9+10): Navigate file operations, from reading and writing to exploring modules and libraries—Discover Python’s built-in modules, including math and random, for enhanced functionality.
Chapter 5: Introduction to Object-Oriented Programming (Page 11): Step into Object-Oriented Programming (OOP). Grasp the essentials—classes, objects, encapsulation, inheritance, and polymorphism—to elevate your coding skills.
Chapter 6: Real-World Applications and Next Steps (Page 12): Witness Python’s real-world impact and explore industry applications. Conclude your beginner’s journey with suggestions for further learning, encouraging exploration into advanced topics.
Introduction
Embark on a captivating journey into the world of Python with our comprehensive beginner’s guide. This book is your key to mastering the fundamentals of programming, demystifying Python’s syntax, and unleashing its potential for diverse applications.
Unravel the Basics: Dive into Python’s core concepts, from variables and data types to control structures, laying a robust foundation for your coding prowess.
Hands-On Learning: Immerse yourself in practical exercises and real-world examples that reinforce your understanding of Python, ensuring a hands-on and engaging learning experience.
Problem-Solving Approach: Explore Python’s problem-solving capabilities through hands-on projects and challenges, enhancing your ability to tackle real-world scenarios.
Beyond the Basics: Move beyond introductory concepts to explore advanced Python features, including functions, modules, and object-oriented programming, equipping you with versatile skills.
Getting Started with Python: A Beginner’s Guide
Welcome to the exciting world of Python! This guide will walk you through downloading, installing, and getting started with Python, a versatile programming language renowned for its simplicity and power.
Step 1: Download Python
Visit the official Python website python.org to access the latest version of Python. Choose the appropriate installer for your operating system (Windows, macOS, or Linux) and follow the download instructions.
Step 2: Installation
Once the download is complete, run the installer. During installation, ensure you check the box that says “Add Python to PATH.” This simplifies running Python commands from the terminal or command prompt.
Step 3: Verify Installation
Open a terminal or command prompt and type python --version
or python -V
to confirm the installation. You should see the installed Python version displayed.
Step 4: Interactive Mode
Explore Python’s interactive mode by typing python
in the terminal. This mode allows you to execute Python commands directly, making it an excellent place for experimentation and learning.
Step 5: Writing Your First Script
Use a text editor (like VSCode, IDLE, or Notepad++ ,pychram) to write a simple Python script. For example:
print(“Hello, Python!”)
Save the file with a .py
extension (e.g., hello.py
).
Step 6: Run Your Script
In the terminal or command prompt, navigate to the directory where your script is saved and type python hello.py
. You should see “Hello, Python!” displayed, marking your first Python script execution.
Try It!!!
Variables:-
- Integer: Represents whole numbers (e.g.,
age = 25
). - Float: Represents numbers with decimal points (e.g.,
temperature = 98.6
). - String: Represents text or characters (e.g.,
name = 'John'
). - Boolean: Represents binary values (
True
orFalse
) (e.g.,is_adult = True
). - List: Ordered collection of items (e.g.,
fruits = ['apple', 'banana', 'orange']
). - Tuple: Similar to a list but immutable (e.g.,
coordinates = (3, 4)
). - Dictionary: Collection of key-value pairs (e.g.,
person = {'name': 'Alice', 'age': 30, 'city': 'New York'}
). - None: Represents the absence of a value or null (e.g.,
result = None
).
Loops, Functions:-
Loops: Unleashing the Power of Repetition
Consider this task: “Write a program that prints ‘9’ a thousand times.”
Your initial instinct might be to repeat the command print('9')
a thousand times, right? But let’s pause for a moment. Do you think this approach truly makes sense?
Absolutely, No!! , Who thinks 🤔 yes.Do not continue learning programming language! 😃
For this reason, there is a loop technique:-
1.) while
2.) for
While Loop: A while
loop in Python repeatedly executes a block of code as long as a specified condition is true. The condition is evaluated before each iteration, and the loop continues until the condition becomes false.
Example:
count = 0
while count < 5:
print(f’Count is {count}’)
count += 1
What do you think, what is the result?
************************************
For Loop: A for
loop is used for iterating over a sequence (that is either a list, tuple, dictionary, string, or other iterable objects). It iterates over each item in the sequence and executes the block of code.
Example:
fruits = [‘apple’, ‘banana’, ‘cherry’]
for fruit in fruits:
print(f’This is a {fruit}’)
What do you think, what is the result?
This chapter is a comprehensive guide to Python’s versatile data structures—lists, tuples, sets, and dictionaries.
- Lists:
- Definition: Ordered, mutable collections.
- Example:
fruits = ['apple', 'banana', 'orange']
- Usage: Ideal for ordered sequences with the potential for modification.
- Tuples:
- Definition: Ordered, immutable collections.
- Example:
coordinates = (3, 4)
- Usage: Suited for fixed data that shouldn’t be altered.
- Sets:
- Definition: Unordered collections of unique elements.
- Example:
unique_numbers = {1, 2, 3, 4}
- Usage: Efficient for membership tests and eliminating duplicates.
- Dictionaries:
- Definition: Unordered collections of key-value pairs.
- Example:
person = {'name': 'Alice', 'age': 30, 'city': 'New York'}
- Usage: Perfect for associating information and quick data retrieval based on keys.
Exercises:
1. List Operations:
- Create a list of your favorite movies.
- Add a new movie to the list.
- Remove the third movie from the list.
- Print the length of the list.
2. Tuple Manipulation:
- Define a tuple with your birthdate (day, month, year).
- Try to change one of the values. What happens?
- Extract and print the year from the tuple.
3. Dictionary Challenges:
- Create a dictionary representing a person (name, age, city).
- Add a new key-value pair for the person’s occupation.
- Print all keys in the dictionary.
- Update the age to reflect a birthday.
4. Combining Structures:
- Create a list of dictionaries, each representing a book (title, author, year).
- Add a new book to the list.
- Print the titles of all books.
5. List Comprehension:
- Generate a list of squares for numbers 1 to 10 using list comprehension.
- Create a list of even numbers from the square list.
6. Nested Structures:
- Create a dictionary of students, where each student has a list of grades.
- Calculate the average grade for each student.
7. Shopping List:
- Create a shopping list dictionary with items as keys and quantities as values.
- Add a new item to the list.
- Remove an item that you’ve already bought.
8. Accessing Elements:
- Given a list
[1, 2, 3, [4, 5, 6], 7]
, print the third element of the nested list.
In this chapter, you’ll delve into the realm of file operations, explore Python’s built-in modules, and harness the power of external libraries. Here’s what you’ll learn:
- File Operations:
- Understand how to read and write files in Python.
- Learn techniques for handling different file formats, such as text and CSV.
- Exploring Modules:
- Discover the concept of modules and how they enhance code organization.
- Learn how to import and use modules in your Python programs.
- Built-in Modules:
- Explore Python’s extensive set of built-in modules.
- Dive into the
math
module for advanced mathematical operations. - Utilize the
random
module for generating random numbers and making simulations.
- External Libraries:
- Gain an introduction to external libraries that extend Python’s capabilities.
- Understand how to install and use external libraries for specific tasks.
- Enhanced Functionality:
- Apply modules and libraries to enhance the functionality of your Python programs.
- See practical examples of how these tools can simplify complex tasks.
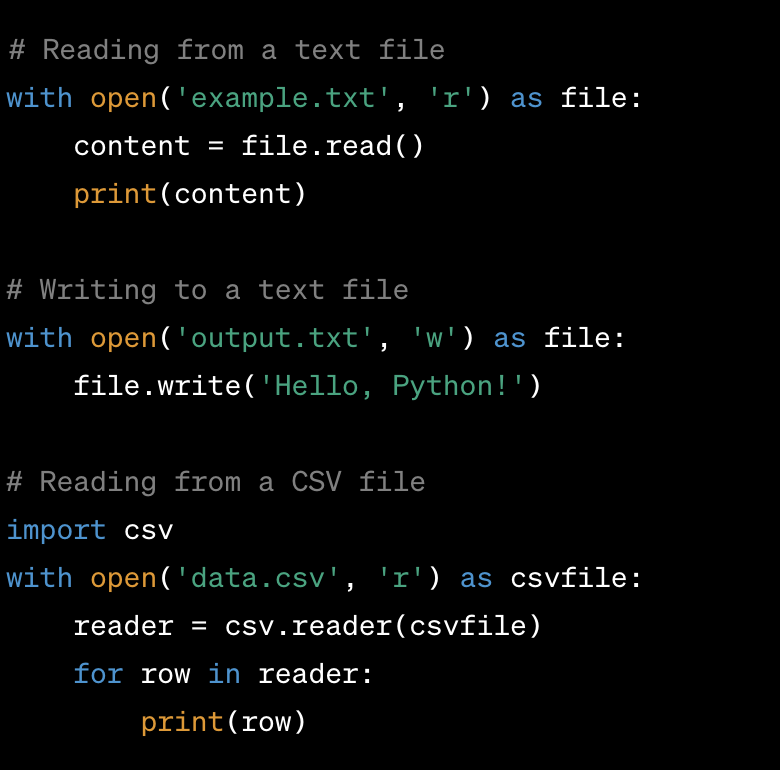
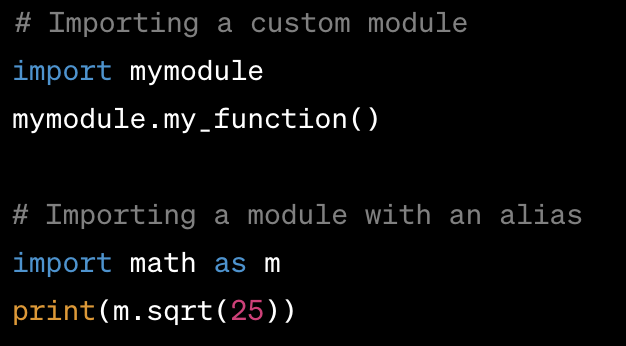
Dear Readers,
Congratulations on completing this journey into the world of Python! I hope you’ve found joy and enlightenment in unraveling the mysteries of programming. Remember, learning is a continuous adventure, and Python is your trusty companion.
As you embark on your coding endeavors, may the principles and skills you’ve acquired here empower you. Special thanks to all the curious minds who joined me on this exploration.
Happy coding!
Mohanad Safi
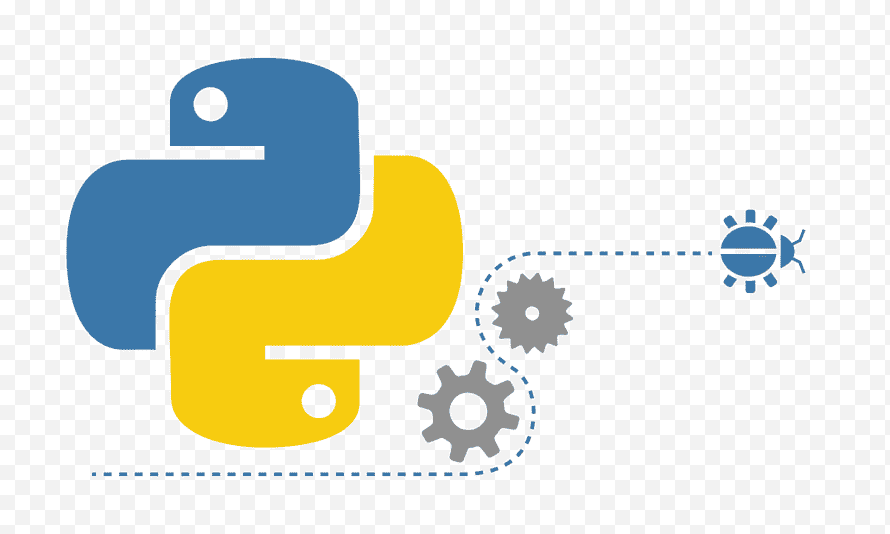
Published: Dec 25, 2023
Latest Revision: Dec 25, 2023
Ourboox Unique Identifier: OB-1532116
Copyright © 2023